DEF CON CTF Qualifier 2023 - Open House (heap)
You are cordially invited to an exclusive Open House event, where dreams meet reality and possibilities abound. Join us for an unforgettable experience as we showcase a captivating array of properties, each waiting to become your dream home.
- [68 solves]
Except simple UAF, my first heap challenge XD
(So the exploit is going to be dirty. :p)
It is very meaningful to me because I exploited the challenge using only Googling.
Analysis
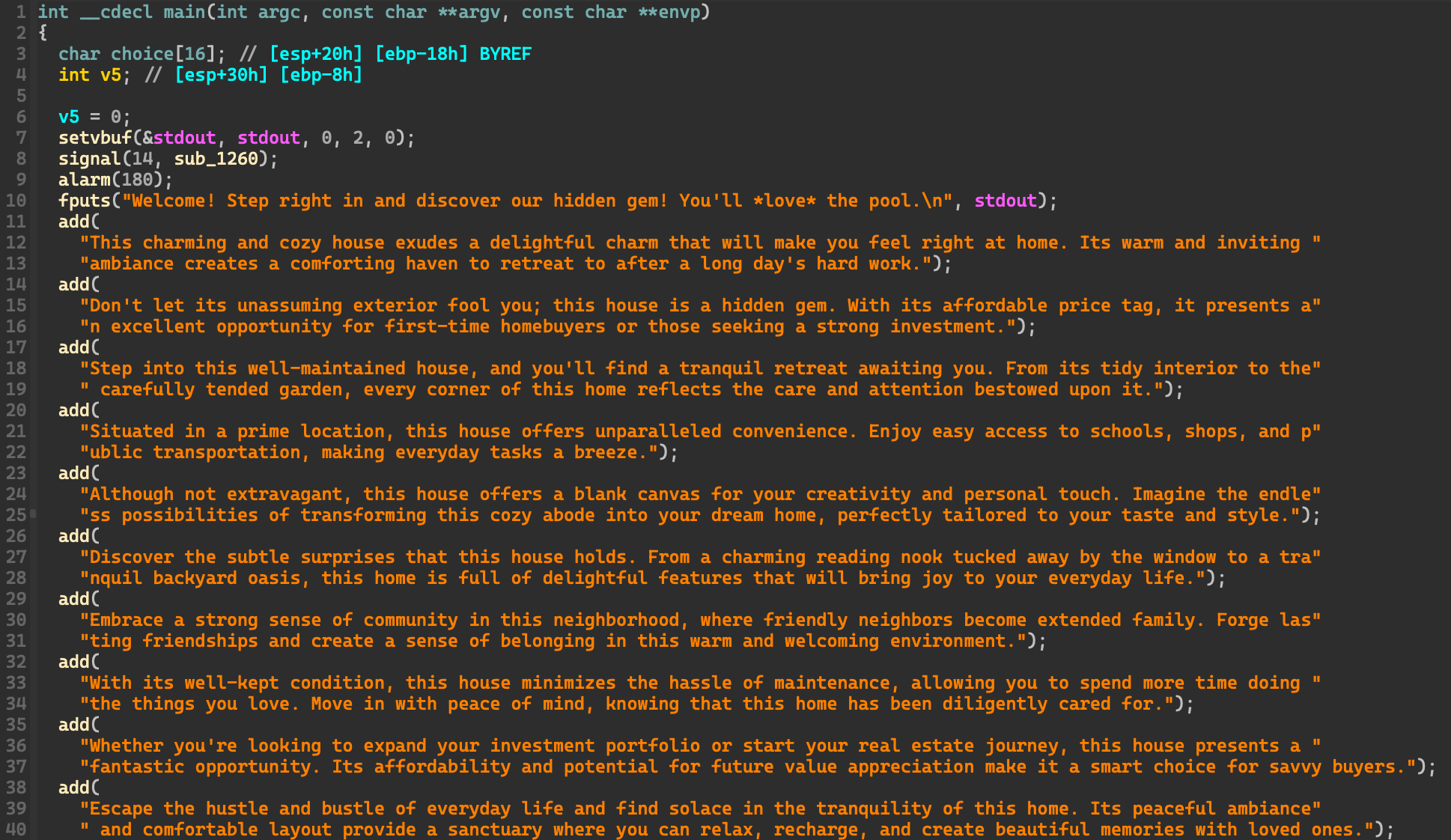
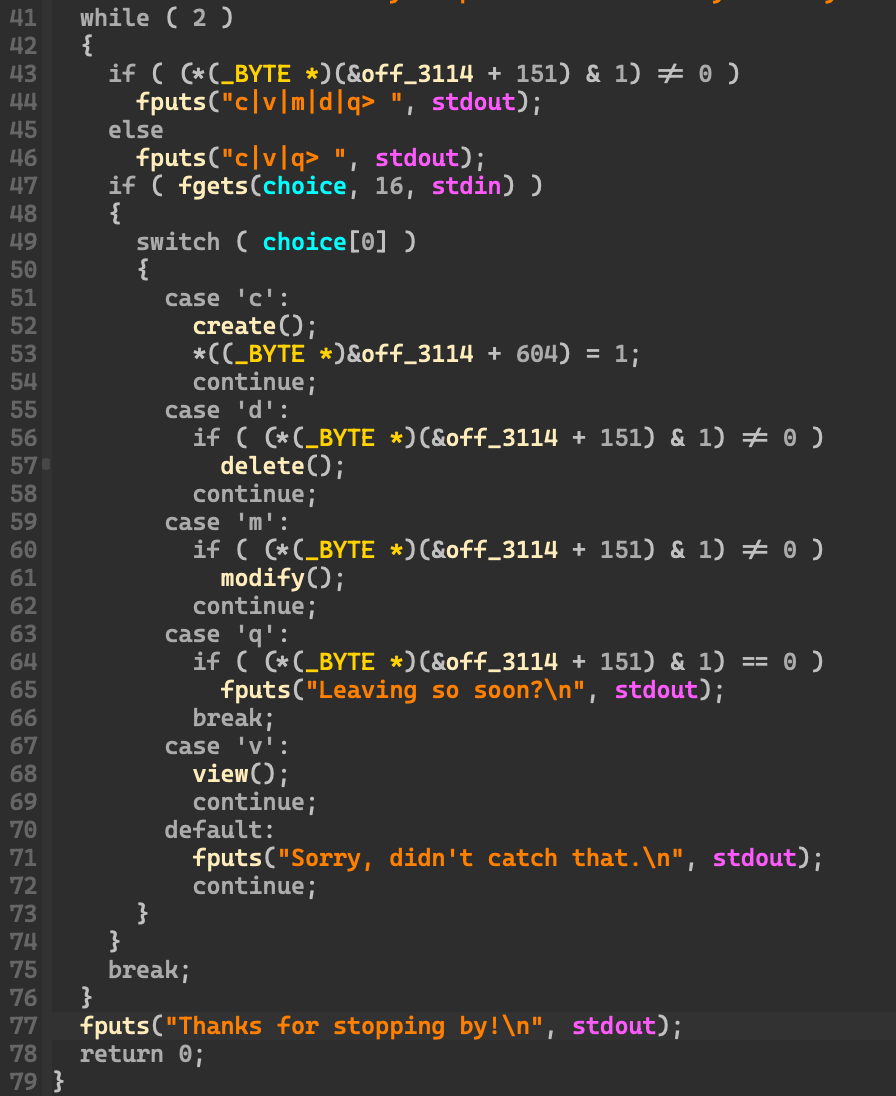
이 프로그램은 4가지의 기능을 가지고 있는데 느낌상 벌써 heap 문제 같다. (네이밍은 다 해준 상태이다.)
This program has 4 functions, and it feels like a heap challenge. (The naming has been done.)
main 함수 앞에서 많이 호출된 add() 함수를 살펴보자. struct를 정의해 주기 전 모습은 정말 복잡했다. 이 함수를 해석하는 데 꽤 오래걸렸다.
Let’s look at the add() function, which is called a lot before the main function. Before defining the struct, it was really complicated. I spent a lot of time interpreting this function.
1 | struct review |
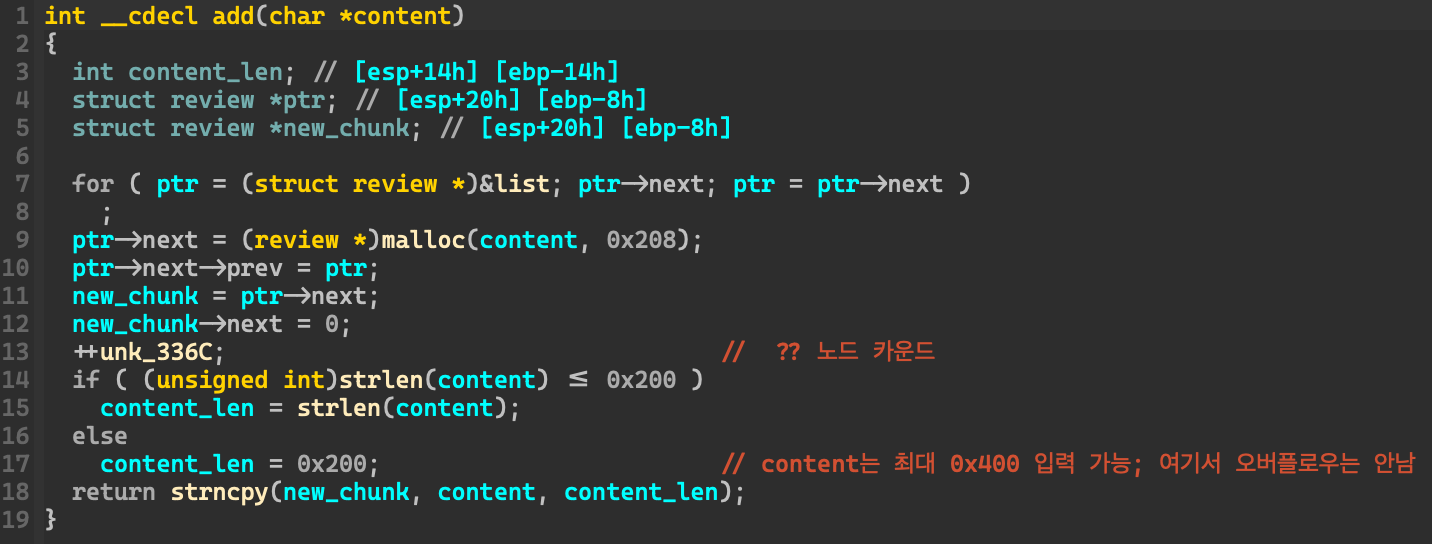
create() 함수에서 content를 입력받고, 인자로 해서 위의 add() 함수를 호출한다. 이때 overflow는 발생하지 않는다. 취약점은 아래 modify() 함수에서 나타난다.
In the create() function, the content is input, and the above add() function is called with the input as a argument. In this case, overflow doesn’t occur. The vulnerability appears in the modify() function below.
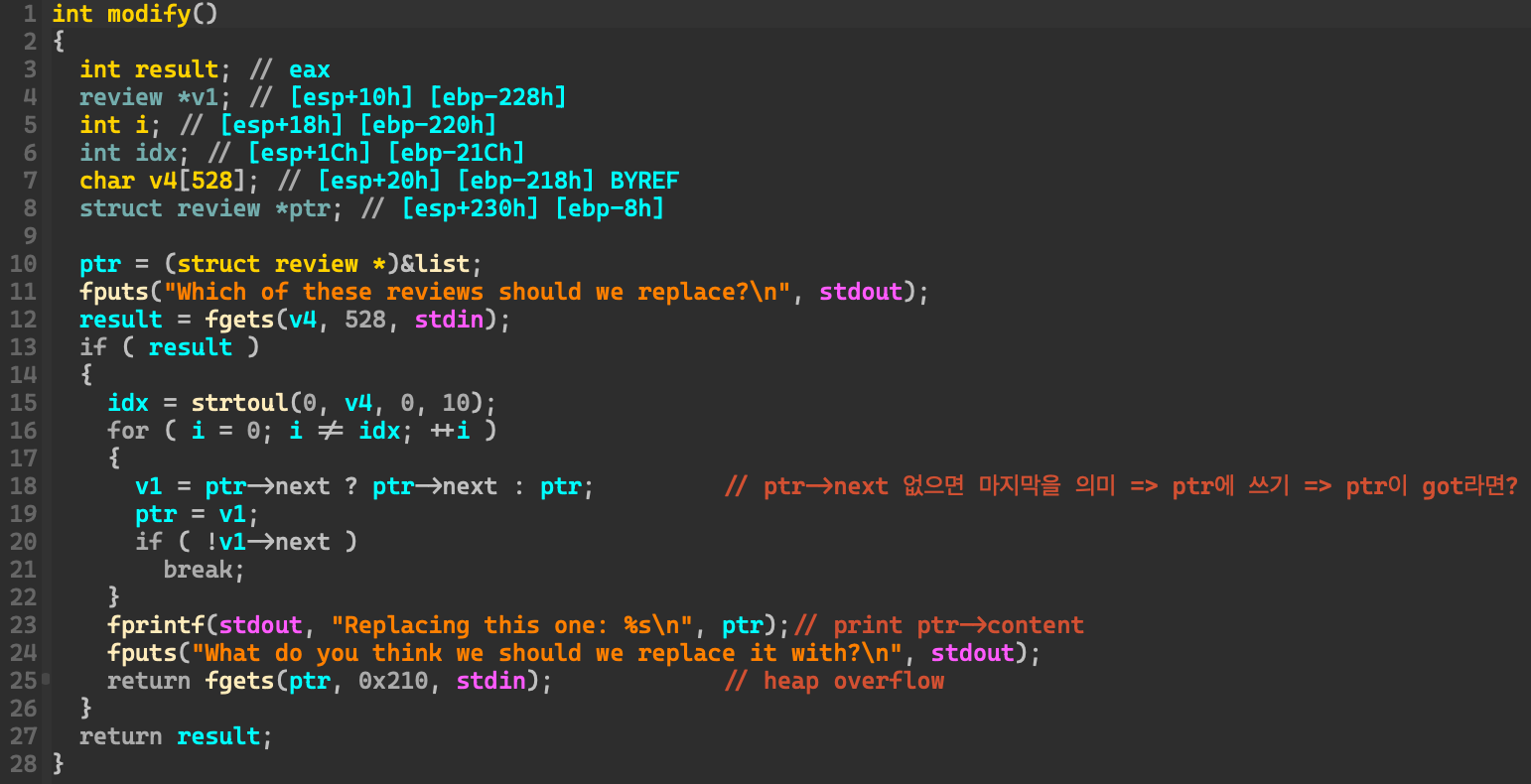
25번째 라인에서 heap overflow가 발생한다. content를 넘어서 next와 prev 포인터까지 덮어쓸 수 있다.
In the 25th lne, heap overflow occurs. You can overwrite the next and prev pointers beyond the content.
Exploit
- libc address 구하기
- 처음에 0x10~0x400이 할당되면 fastbin이나 unsorted bin에 들어가지 않고 tcache bin에 들어간다.
- unsorted bin의 특성을 이용해서 구할 수 있다. 하지만 tcache가 존재하기 때문에 tcache bin이 가득 차고 난 후, unsorted bin에 청크가 저장된다.
- 8개를 free하고, 9번째부터 unsorted bin에 저장된다.
- 이때 청크에 libc 주소가 적혀있다. 이것을 릭하였다.
- 이 블로그를 참고하였음: https://velog.io/@woounnan/SYSTEM-Heap-Basics-Bin
- libc.symbols[‘environ’]에 저장된 stack address 구하기
- pie base address를 구하기 위해 stack address를 구했다.
- pie base address 구하기
- stack에는 높은 확률로 pie address가 존재한다.
- e.got[‘strlen’]을 system 함수로 덮음
- create() 함수에서 strlen(입력) 형태로 사용하기 때문에 strlen을 system 함수로 덮고 입력으로 ‘/bin/sh’를 보냈다.
- Getting libc address
- If 0x10~0x400 is initially allocated, it goes into tcache bin instead of fastbin or unsorted bin.
- It can be caculated by using the characteristics of unsorted bins. But because tcache exists, tcache bin is full and then chunks are stored in unsorted bin.
- 8 are freed, and from the 9th are stored in the unsorted bin.
- At this time, the libc address is written in the chunk. I leaked this.
- Referred to this blog(Korean): https://velog.io/@woounnan/SYSTEM-Heap-Basics-Bin
- Getting the stack address stored in libc.symbols[‘environ’]
- The stack address was leaked to get the pie base address.
- Getting the pie base address
- There is a pie address in the stack with a high probability.
- Overwriting e.got[‘strlen’] with the system function
- Since strlen(input) is used in the create() function, strlen is overwritten by the system function and ‘/bin/sh’ is sent as an input.
중간 중간 leak하면서 프로그램이 자꾸 종료되었다. 그래서 아래 payload를 이용하여 next 포인터를 정상적으로 만들어주고 다음 leak을 진행하였다.
The program exit after leak. So, by using the payload below, I made next pointer normally and proceeded with the next leak.
1 | payload = b'A' * (0x200-1) + b'B' |
Exploit Code
1 | from pwn import * |