3st stage MetaRed CTF 2023 - Master JWT (web)
With just two requests you can get the flag. Read the code :) Don’t brute-force paths, you have the source code uploaded.
- [48 solves / 401 points]
I was toooo happy to solve this web challenge!!
Analysis
This is the source code provided.
1 | from flask import Flask, request, jsonify, make_response |
The server gets the Authorization
value from the header of the request. If it doesn’t exist, the server creates and send to response JWT token using app.secret_key
and HS256
algorithm.
If Authorization
value is exit, the server will try to decode it using app.secret_key
and HS256
algorithm and obtain the user
value. If the user
value is admin
, you can get the flag!
An structure of a JWT is shown below:
1 | xxxx.yyyy.zzzz |
I had doubts. Can I guess the input(JWT token) from the response? Can I know the app.secret_key
?
So, I tried below things for my doubts.
- using
None
algorithm instead ofHS256
when sending jwt token.- It was wrong. Because it’s fixed the value of
algorithms=['HS256']
when decoding. - I could have tried this if the algorithm wasn’t fixed.
- It was wrong. Because it’s fixed the value of
- try to find
app.secret_key
.1
2
3# app.py
random.seed(f"Sup3S4f3{randint(0, 1000)}")
app.secret_key = f"Sup3S4f3{randint(0, 1000000000000)}"- If I know the seed, I can know the random value. It’s not completely random. The number of cases is only 1001.
- I used the hashcat to find the
key
of jwt token created using theHS256
algorithm. For this, I needed to write a script to create the key candidate list.1
2
3
4
5
6import random
from random import randint
for i in range(1001):
random.seed(f"Sup3S4f3{i}")
print(f"Sup3S4f3{randint(0, 1000000000000)}")
Finally I got the list for hashcat!
- https://github.com/hashcat/hashcat
1
hashcat -m 16500 eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VyIjoibm9uIHByaXZpbGVnZWQifQ.SmQA4Ic4hOGFRD4hdRuuact9gwKRZZwI3zpEgBnjocw ./list
The -m
means hash modes, 16500 is JWT (JSON Web Token). Now I know the app.secret_key
from the result. It was Sup3S4f3472520232207
.
I used this site for create jwt token.
Solve
- Just connect and receive a jwt token in response.
- Infer the key used for encoding using the obtained jwt token and hashcat.
- Change the
user
value to admin, create a new jwt token, and send it.
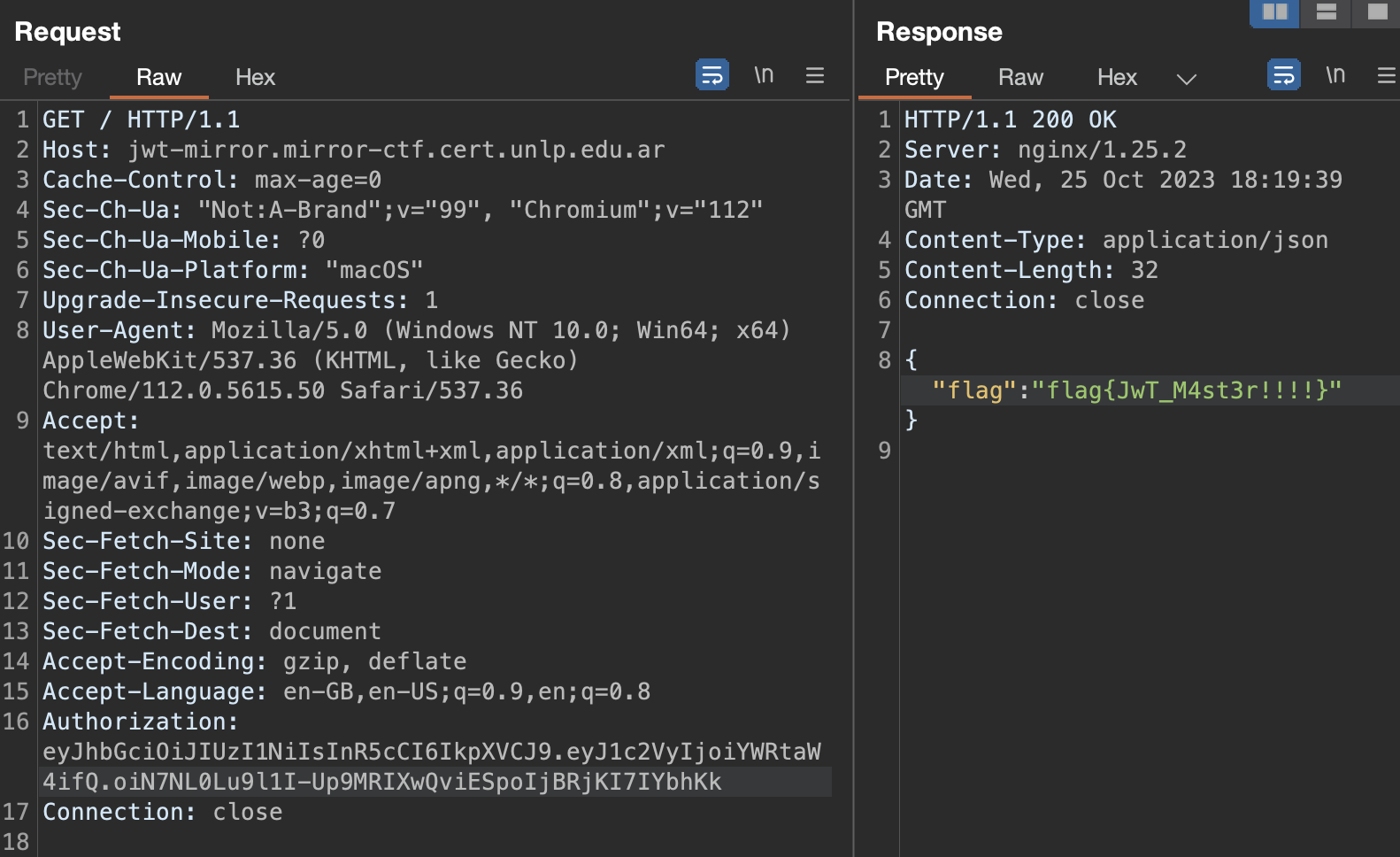